Learning | Hello World in different Languages
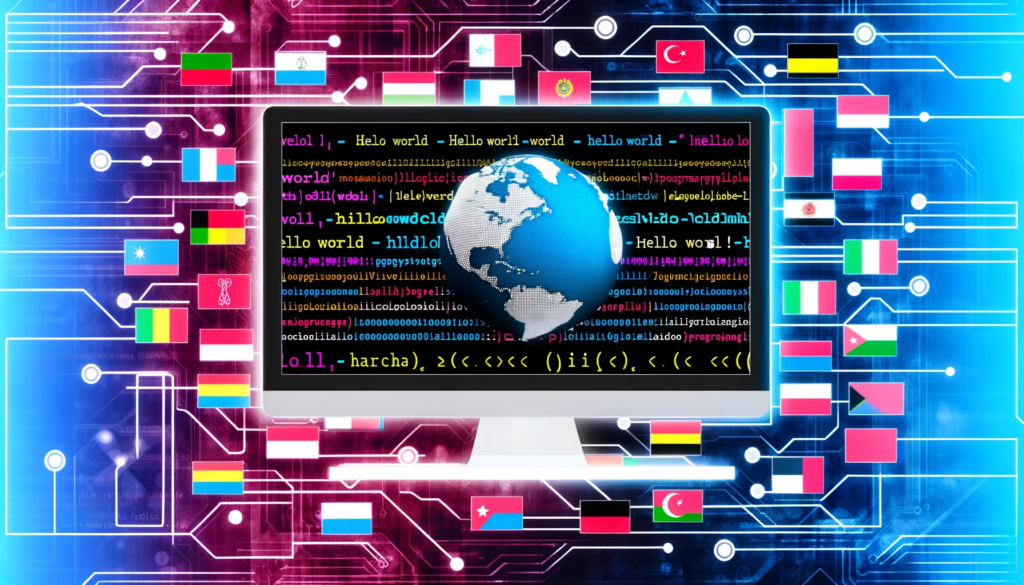
Python
print("Hello, World!")
Java
public class HelloWorld { public static void main(String[] args) { System out println("Hello, World!"); } }
C
#include <stdio h> int main() { printf("Hello, World!\n"); return 0; }
4 C++:
#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
JavaScript
console log("Hello, World!");
Ruby
puts "Hello, World!"
Swift
print("Hello, World!")
Go
package main import "fmt" func main() { fmt Println("Hello, World!") }
Rust
fn main() { println!("Hello, World!"); }
PHP
<?php echo "Hello, World!"; ?>
Perl
print "Hello, World!\n";
Kotlin
fun main() { println("Hello, World!") }
Scala
object HelloWorld { def main(args: Array[String]): Unit = { println("Hello, World!") } }
Lua
print("Hello, World!")
Haskell
main :: IO () main = putStrLn "Hello, World!"
Dart
void main() { print('Hello, World!'); }
Shell
echo "Hello, World!"
Batch
@echo off echo Hello, World!
PowerShell
Write-Output "Hello, World!"
VBScript
MsgBox "Hello, World!"
Objective-C
#import <Foundation/Foundation h> int main(int argc, const char * argv[]) { @autoreleasepool { NSLog(@"Hello, World!"); } return 0; }
Assembly
section data hello db 'Hello, World!',10 len equ $ - hello section text global _start _start: ; write our string to stdout mov eax, 4 ; sys_write mov ebx, 1 ; file descriptor 1 (stdout) mov ecx, hello ; message to write mov edx, len ; message length int 0x80 ; syscall ; exit mov eax, 1 ; sys_exit xor ebx, ebx ; exit status 0 int 0x80 ; syscall
VBA (Visual Basic for Applications)
Sub HelloWorld() MsgBox "Hello, World!" End Sub
Tcl
puts "Hello, World!"
COBOL
IDENTIFICATION DIVISION PROGRAM-ID HELLO-WORLD PROCEDURE DIVISION DISPLAY "Hello, World!" STOP RUN
26 F#:
printfn "Hello, World!"
Elixir
IO puts "Hello, World!"
SQL (MySQL)
SELECT 'Hello, World!';
SQL (SQLite)
SELECT 'Hello, World!';
SQL (PostgreSQL)
SELECT 'Hello, World!';
SQL (Oracle)
SELECT 'Hello, World!' FROM DUAL;
SQL (SQL Server)
PRINT 'Hello, World!';
Smalltalk
Transcript show: 'Hello, World!'; cr
R
cat("Hello, World!\n")
Bash
echo "Hello, World!"
Erlang
-module(hello) -export([hello_world/0]) hello_world() -> io:fwrite("Hello, World!~n")
Julia
println("Hello, World!")
MATLAB
disp('Hello, World!');
AutoHotkey
MsgBox, Hello, World!
Clojure
(println "Hello, World!")
Groovy
println "Hello, World!"
OCaml
print_endline "Hello, World!"
D
import std stdio; void main() { writeln("Hello, World!"); }
Crystal
puts "Hello, World!"
Nim
echo "Hello, World!"
Common Lisp
(format t "Hello, World!Scheme
(display "Hello, World!") (newline)Prolog
:- initialization(main) main :- write('Hello, World!'), nl, haltABAP
REPORT ZHELLO_WORLD WRITE: / 'Hello, World!'VB NET
vb net Module HelloWorld Sub Main() Console WriteLine("Hello, World!") End Sub End Module